OpenGL应用框架-GLFW


GLFW是一个OpenGL的应用框架,支持Linux和Windows。主要用来处理特定操作系统下的特定任务,例如 OpenGL 窗口管理、分辨率切换、键盘、鼠标以及游戏手柄、定时器输入、线程创建等等。GLFW 是配合 OpenGL 使用的轻量级工具程序库,缩写自 Graphics Library FrameWork(图形库框架)。其主要功能是创建并管理窗口和 OpenGL 上下文,支持OpenGL、ES、Vulkan,支持多窗口高DPI和gamma ramps,支持常用外设。有类似功能的库还有 GLUT 和 SDL,是继GLUT,FreeGLUT之后,当前最新版本可用来创建OpenGL上下文,以及操作窗口的第三方开发库。
GLFW is an Open Source, multi-platform library for creating windows with OpenGL contexts and managing input and events. It is easy to integrate into existing applications and does not lay claim to the main loop.
在计算机图形学和游戏开发领域,GLFW(Graphics Library Framework)库是一个广受欢迎的开源库,它为开发者提供了创建和管理窗口、处理输入事件以及与 OpenGL、Vulkan 等图形 API 交互的便捷方式。其最初由 Marcus Geelnard 于 2002 年开发,旨在为 OpenGL 应用程序提供一个简单的跨平台窗口创建和事件处理框架。经过多年的发展,其已经成为一个功能强大、稳定且易于使用的库,支持 Windows、macOS、Linux 等多种主流操作系统。
主要特性
跨平台支持:GLFW 能够在不同的操作系统上运行,使得开发者可以编写一次代码,在多个平台上部署应用程序,大大提高了开发效率。
窗口管理:GLFW 提供了一系列函数来创建、销毁和管理窗口,包括设置窗口大小、位置、标题、图标等属性,还支持全屏模式和多显示器环境。
输入事件处理:GLFW 可以捕获键盘、鼠标、游戏手柄等输入设备的事件,如按键按下、释放、移动、滚轮滚动等,方便开发者实现交互功能。
OpenGL 和 Vulkan 集成:GLFW 与 OpenGL 和 Vulkan 图形 API 紧密集成,能够方便地创建上下文并进行图形渲染,无论是 2D 还是 3D 图形开发都能轻松应对。
高分辨率支持:支持高分辨率显示器,确保在 Retina 屏幕等设备上能够提供清晰、细腻的图像显示。
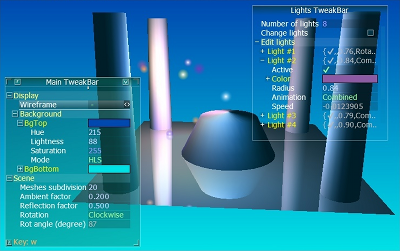
GLFW is written in C and has native support for Windows, Mac OS X and many Unix-like systems using the X Window System, such as Linux and FreeBSD. Licensed under the zlib/libpng license.
Features
Easy to use API in the style of the OpenGL APIs
Gives you a window and OpenGL context with two function calls
Explicit support for MSAA, sRGB, robustness, OpenGL 3.2+ and OpenGL ES
Support for multiple monitors, high-DPI, gamma ramps and clipboard
Input can be either polled or delivered via callbacks
UTF-8 for all strings and basic Unicode character input
Supports both static and dynamic linking
Open Source with a liberal OSI-certified license
Examples and comprehensive documentation
使用方法
在使用 GLFW 库之前,需要先将其安装到开发环境中。可以从 GLFW 官方网站下载源代码进行编译安装,也可以使用包管理器(如在 Linux 上使用 apt-get 或 yum,在 Windows 上使用 vcpkg)进行安装。基本用法如下:
使用 GLFW 库创建一个简单的窗口并显示,一般需要以下几个步骤:
1.初始化 GLFW 库:调用glfwInit()函数进行初始化。
2.创建窗口:使用glfwCreateWindow()函数创建一个窗口,并设置其大小、标题等属性。
3.创建 OpenGL 上下文:通过glfwMakeContextCurrent()函数将窗口的上下文设置为当前上下文。
4.事件循环:在一个循环中不断处理输入事件并进行渲染,直到用户关闭窗口。
5.清理资源:在程序结束时,调用glfwTerminate()函数释放 GLFW 库占用的资源。
应用场景
游戏开发:GLFW 库为游戏开发者提供了一个快速搭建游戏窗口和处理用户输入的基础框架,结合 OpenGL 或 Vulkan 等图形 API,可以创建出高性能的 2D 和 3D 游戏。
图形学研究:在计算机图形学的教学和研究中,GLFW 常用于创建图形演示程序,帮助学生和研究人员理解和实现各种图形算法。
虚拟现实(VR)和增强现实(AR)开发:GLFW 对多显示器和高分辨率的支持,使其成为 VR 和 AR 应用开发的理想选择,能够为用户提供沉浸式的体验。
GLFW 库以其简洁易用、功能强大和跨平台的特性,成为了图形开发领域不可或缺的工具。无论是初学者还是经验丰富的开发者,都可以通过 GLFW 库快速实现自己的图形应用程序,探索计算机图形学的无限可能。随着技术的不断发展,相信 GLFW 库会在更多的领域发挥重要作用。
GLAD
因为OpenGL只是一个标准/规范,具体的实现是由驱动开发商针对特定显卡实现的。由于OpenGL驱动版本众多,它大多数函数的位置都无法在编译时确定下来,需要在运行时查询。所以任务就落在了开发者身上,开发者需要在运行时获取函数地址并将其保存在一个函数指针中供以后使用,取得地址的方法因平台而异。
Vulkan/GL/GLES/EGL/GLX/WGL Loader-Generator based on the official specifications for multiple languages.
GLAD是一个开源的库,它能解决我们上面提到的那个平台问题。其配置与大多数的开源库有些许的不同,GLAD使用了一个在线服务。在这里能够告诉GLAD需要定义的OpenGL版本,并且根据这个版本加载所有相关的OpenGL函数。在其在线服务页面中将语言(Language)设置为C/C++,在API选项中,选择3.3以上的OpenGL(gl)版本(不少教程中将使用3.3版本,但更新的版本也能用)。之后将模式(Profile)设置为Core,并且保证选中了生成加载器(Generate a loader)选项。现在可以先(暂时)忽略扩展(Extensions)中的内容。都选择完之后,点击生成(Generate)按钮来生成库文件。稍后会应该提供给了一个zip压缩文件,包含两个头文件目录,和一个glad.c文件。将两个头文件目录(glad和KHR)复制到你的Include文件夹中(或者增加一个额外的项目指向这些目录),并添加glad.c文件到你的工程中。
经过前面的这些步骤之后,你就应该可以将以下的指令加到你的文件顶部了:
#include <glad/glad.h>
可以阅读Getting started来理解GLFW3的使用,glfw/examples目录下有数十个例子可以参考。
使用步骤:
首先包含头头文件
#include <glad/glad.h>
// GLFW (include after glad)
#include <GLFW/glfw3.h>
初始化glfw库:
在glfw使用之前先要出世,初始化只要是检查环境是不是支持。
GLFWAPI int glfwInit(void);
初始化GLFW库,在应用称许执行之前要先调用这个函数,对应的终止函数是glfwTerminate,在应用程序结束之前要调用glfwTerminate,成功返回GLFW_TRUE
设置错误回调:
GLFWAPI GLFWerrorfun glfwSetErrorCallback(GLFWerrorfun callback);
设置错误回调函数,当一个错误发生时回调用错误码和一段描述信息。回调函数的个是如下:
void error_callback(int error, const char* description){
fprintf(stderr, "Error: %s\n", description);
}
创建窗口和上下文:
GLFWAPI void glfwWindowHint(int hint, int value);
GLFWAPI void glfwWindowHintString(int hint, const char* value);
设置window的hints值,在glfwCreatewindow时生效,设置之后不会改变,知道遇到函数glfwDefaultWindowHints或GLFW终止。这个函数只能设置整形值,字符串的值通过glfwWindowHintString来设置。另外它不检查设置的值是否有效,在glfwCreateWindow的时候才会报。
GLFWAPI GLFWwindow* glfwCreateWindow(int width, int height, const char* title, GLFWmonitor* monitor, GLFWwindow* share);
创建GL/ES的上下文,参数指定了上下文如何创建,成功创建不影响当前上下文。
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 2);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 0);
GLFWwindow* window = glfwCreateWindow(640, 480, "My Title", NULL, NULL);
if (!window){
// Window or context creation failed
}
设置上下文:
GLFWAPI void glfwMakeContextCurrent(GLFWwindow* window);
指定当前GL/ES上下文
检查窗口的关闭标识:
GLFWAPI int glfwWindowShouldClose(GLFWwindow* window);
检查指定window的关闭状态.
while ( glfwWindowShouldClose(window) ){
//keep running
}
接收输入事件:
先设置事件回调函数,输入事件比较多。
static void key_callback(GLFWwindow* window, int key, int scancode, int action, int mods){
if (key == GLFW_KEY_ESCAPE && action == GLFW_PRESS)
glfwSetWindowShouldClose(window, GLFW_TRUE);
}
glfwSetKeyCallback(window, key_callback);
进行渲染:
渲染的时候framebuffer的size可以设置和viewport一样大,也可以设置framebuffer size的回调函数。
int width, height;
glfwGetFramebufferSize(window, &width, &height);
glViewport(0, 0, width, height);
时间读取:
GLFWAPI double glfwGetTime(void);
获取GLFW的时间,以秒为单位
GLFWAPI void glfwSetTime(double time);
设置GLFW的时间,以秒为单位,小于等于18446744073.0
GLFWAPI uint64_t glfwGetTimerValue(void);
获取raw timer的值,单位1 / frequency seconds
GLFWAPI uint64_t glfwGetTimerFrequency(void);
获取帧率单位是Hz
缓冲区交换:
GLFWAPI void glfwSwapBuffers(GLFWwindow* window);
交换窗口的前后缓冲区,如果交换间隔大于0,交换缓冲区之前GPU驱动会等待specified number of screen updates
GLFWAPI void glfwSwapInterval(int interval);
设置当前上下文的交换间隔
事件处理:
有两种处理未决事件的方法; 轮询和等待。
GLFWAPI void glfwPollEvents(void);
处理所有事件队列中的事件,事件处理会调用一些回调函数。一些平台下,窗口移动、resize和菜单操作都会触发事件处理,block当前的任务。
GLFWAPI void glfwWaitEvents(void);
等待所有处理中和队列中的事件完成,当前线程会进入sleep状态,如果有事件可用,就相当与glfwPollEvents.
销毁和注销:
GLFWAPI void glfwTerminate(void);
这个函数终止GLFW,这个函数会注销调剩余的windows and cursors,复位所有修改过的gamma ramps,释放申请的资源。对应glfwInit
其他API
GLFWAPI void glfwInitHint(int hint, int value);
设置制定的初始化hint值,这个设置之后中间不能进行修改直至结束。有些hints是平台相关,这些只有在对应的平台上才会生效。
GLFWAPI void glfwGetVersion(int* major, int* minor, int* rev);
查询GLFW库的版本,major, minor and revision号
GLFWAPI const char* glfwGetVersionString(void);
查询编译阶段的配置,返回的是个字符串,包含版本、平台、编译器和平台相关的编译阶段的选项
GLFWAPI int glfwGetError(const char** description);
查询错误码并清除上一次的错误信息
GLFWAPI GLFWmonitor** glfwGetMonitors(int* count);
查询当前的连接监视器connected monitors,返回的是当前所有连接的monitor,primary monitor永远是数组的第一个。
GLFWAPI GLFWmonitor* glfwGetPrimaryMonitor(void);
查询primary monitor,task bar和global menu bar等基本信息都是显示在primary monitor中的。
GLFWAPI void glfwGetMonitorPos(GLFWmonitor* monitor, int* xpos, int* ypos);
获取虚拟屏幕中monitor的视口坐标
GLFWAPI void glfwGetMonitorWorkarea(GLFWmonitor* monitor, int* xpos, int* ypos, int* width, int* height);
获取monitor的工作区,返回的是屏幕坐标的位置,左上角和size的值。工作区是屏幕中没有系统任务条的区域,如果系统没有任务条,就是全屏幕。
GLFWAPI void glfwGetMonitorPhysicalSize(GLFWmonitor* monitor, int* widthMM, int* heightMM);
获取monitor的物理尺寸,单位是millimetres
GLFWAPI void glfwGetMonitorContentScale(GLFWmonitor* monitor, float* xscale, float* yscale);
获取monitor的content scale,这个值的计算:当前DPI与平台默认DPI之间的比。依赖于monitor的分辨率和设置的像素密度。
GLFWAPI const char* glfwGetMonitorName(GLFWmonitor* monitor);
获取monitor的名称
GLFWAPI void glfwSetMonitorUserPointer(GLFWmonitor* monitor, void* pointer);
设置monitor中用户自定义的pointer
GLFWAPI void* glfwGetMonitorUserPointer(GLFWmonitor* monitor);
获取monitor的用户自定义pointer
GLFWAPI const GLFWvidmode* glfwGetVideoModes(GLFWmonitor* monitor, int* count);
获取monitor的可用视频模式,返回的是一个数组,按照升序排列。
GLFWAPI const GLFWvidmode* glfwGetVideoMode(GLFWmonitor* monitor);
获取monitor的当前的视频模式,如果是全屏模式,返回值以来窗口是否iconified
GLFWAPI void glfwSetGamma(GLFWmonitor* monitor, float gamma);
根据指定的exponent生成一个合适尺寸的gamma ramp(gamma斜度)并设置,参数必须是一个大于0的finite number,用于色彩矫正,理想值是1.
GLFWAPI const GLFWgammaramp* glfwGetGammaRamp(GLFWmonitor* monitor);
获取当前的gamma ramp值
GLFWAPI void glfwSetGammaRamp(GLFWmonitor* monitor, const GLFWgammaramp* ramp);
设置monitor的gamma ramp,第一次调用这个函数并存储gamma的值是在glfwTerminate函数中
GLFWAPI void glfwDefaultWindowHints(void);
reset窗口的所有hints值,全设置为默认的。
GLFWAPI void glfwDestroyWindow(GLFWwindow* window);
destory指定的window和context
GLFWAPI void glfwSetWindowShouldClose(GLFWwindow* window, int value);
设置window的close flag。
GLFWAPI void glfwSetWindowTitle(GLFWwindow* window, const char* title);
设置窗口的title
GLFWAPI void glfwSetWindowIcon(GLFWwindow* window, int count, const GLFWimage* images);
为窗口设置Icon,传入一组备选图像,会选择尺寸接近的作为图标,如果没有传图像,用默认的图标。图像是32bit小端non-premultiplied RGBA8,按行排列从左上角开始。图像的尺寸会根据平台和系统设置进行resize,一般包括16x16,32x32和48x48.
GLFWAPI void glfwGetWindowPos(GLFWwindow* window, int* xpos, int* ypos);
获取指定区域的位置,返回的是屏幕坐标,原点在左上角
GLFWAPI void glfwSetWindowPos(GLFWwindow* window, int xpos, int ypos);
设置窗口坐标,如果是全屏没有效果
GLFWAPI void glfwGetWindowSize(GLFWwindow* window, int* width, int* height);
获取窗口的size,以屏幕坐标为单位,不是framebuffer的尺寸。
GLFWAPI void glfwSetWindowSizeLimits(GLFWwindow* window, int minwidth, int minheight, int maxwidth, int maxheight);
设置窗口的尺寸限制,如果是全屏模式,这个参数只有在创建窗口的时候其作用。其他情况下窗口要是可缩放的这个函数才有作用。
GLFWAPI void glfwSetWindowAspectRatio(GLFWwindow* window, int numer, int denom);
设置窗口的aspect ratio,如果是全屏模式,这个参数只有在创建窗口的时候其作用。其他情况下窗口要是可缩放的这个函数才有作用。aspect ratios是长宽比要大于0。
GLFWAPI void glfwSetWindowSize(GLFWwindow* window, int width, int height);
设置窗口的size.对于全屏窗口,此功能可更新其所需视频模式的分辨率并切换到最接近其的视频模式,而不会影响窗口的上下文。 由于上下文不受影响,因此帧缓冲区的位深度保持不变.
GLFWAPI void glfwGetFramebufferSize(GLFWwindow* window, int* width, int* height);
获取窗口的framebuffer的zise,以pixel为单位
GLFWAPI void glfwGetWindowFrameSize(GLFWwindow* window, int* left, int* top, int* right, int* bottom);
以屏幕坐标为单位检索指定窗口框架的每个边缘的大小。包括标题栏。函数检索每个窗框边缘的大小,而不是沿特定坐标轴的偏移量,所以检索到的值将始终为零或正数。
GLFWAPI void glfwGetWindowContentScale(GLFWwindow* window, float* xscale, float* yscale);
此函数检索指定窗口的内容比例。 内容比例是当前DPI与平台默认DPI之比。 这对于文本和任何UI元素尤其重要。
GLFWAPI float glfwGetWindowOpacity(GLFWwindow* window);
获取窗苦的不透明度(alpha值),包括所有装饰物。该值时0到1之间的值,初始值为1
GLFWAPI void glfwSetWindowOpacity(GLFWwindow* window, float opacity);
设置窗口的透明度
GLFWAPI void glfwIconifyWindow(GLFWwindow* window);
如果指定的窗口先前已还原,则此功能可图标化(最小化)指定的窗口。如果窗口已被图标化,则此功能不执行任何操作。如果指定的窗口是全屏窗口,则将还原原始监视器分辨率,直到还原该窗口为止。
GLFWAPI void glfwRestoreWindow(GLFWwindow* window);
重置窗口,用在窗口进行了最大化或最小化的情况。如果指定的窗口是全屏窗口,则为该窗口选择的分辨率将在所选监视器上恢复。
GLFWAPI void glfwMaximizeWindow(GLFWwindow* window);
最大化窗口,如果窗口已经最大化,或是全屏模式不起作用
GLFWAPI void glfwShowWindow(GLFWwindow* window);
显示窗口,全屏不起作用,默认情况所有创建的窗口都是显示的,也可以通过glfwSetWindowAttrib设置GLFW_FOCUS_ON_SHOW_hint来设置
GLFWAPI void glfwHideWindow(GLFWwindow* window);
隐藏窗口,全屏不起作用
GLFWAPI void glfwFocusWindow(GLFWwindow* window);
此功能将指定的窗口置于最前面并设置输入焦点。 该窗口应该已经可见并且没有图标。默认情况下,窗口和全屏模式窗口在最初创建时都会被聚焦。GLFWAPI void glfwRequestWindowAttention(GLFWwindow* window);
该功能要求用户注意指定的窗口。 作用不详
GLFWAPI GLFWmonitor* glfwGetWindowMonitor(GLFWwindow* window);
返回指定窗口处于全屏状态的监视器的句柄
GLFWAPI void glfwSetWindowMonitor(GLFWwindow* window, GLFWmonitor* monitor, int xpos, int ypos, int width, int height, int refreshRate);
设置指定窗口处于全屏状态的监视器的句柄,这个函数会更新monitor的宽、高、视频模式的刷新率。
GLFWAPI int glfwGetWindowAttrib(GLFWwindow* window, int attrib);
获取窗口或GL/ES上下文相关的属性值,framebuffer相关的hints不是属性。
GLFWAPI void glfwSetWindowAttrib(GLFWwindow* window, int attrib, int value);
设置窗口的属性值,支持的属性包含:GLFW_DECORATED、GLFW_RESIZABLE、GLFW_FLOATING、GLFW_AUTO_ICONIFY、GLFW_FOCUS_ON_SHOW。
GLFWAPI void glfwSetWindowUserPointer(GLFWwindow* window, void* pointer);
设置window的user pointer
GLFWAPI void* glfwGetWindowUserPointer(GLFWwindow* window);
返回window的user pointer
GLFWAPI void glfwWaitEventsTimeout(double timeout);
等待超时。
GLFWAPI void glfwPostEmptyEvent(void);
向事件队列中插入一个空事件
GLFWAPI int glfwGetInputMode(GLFWwindow* window, int mode);
获取窗口输入选项的值,mode取值为:GLFW_CURSOR, GLFW_STICKY_KEYS, GLFW_STICKY_MOUSE_BUTTONS, GLFW_LOCK_KEY_MODS, GLFW_RAW_MOUSE_MOTION。
GLFWAPI void glfwSetInputMode(GLFWwindow* window, int mode, int value);
设置窗口的输入模式,mode取值和get函数一样。
GLFWAPI int glfwRawMouseMotionSupported(void);
查询当前系统是否支持raw mouse motion,是否支持在初始化GLFW的时候一次确定,raw motion接近actual motion,它不受应用于桌面光标运动的缩放和加速度的影响。 该处理适合于光标,而原始运动更适合于控制3D相机。因此,仅在禁用光标时才提供原始鼠标移动。
GLFWAPI const char* glfwGetKeyName(int key, int scancode);
获取按键名。
GLFWAPI int glfwGetKeyScancode(int key);
获取按键码。
GLFWAPI int glfwGetKey(GLFWwindow* window, int key);
获取按键的最后一个状态。
GLFWAPI int glfwGetMouseButton(GLFWwindow* window, int button);
获取鼠标的最后状态值。
GLFWAPI void glfwGetCursorPos(GLFWwindow* window, double* xpos, double* ypos);
获取光标位置,屏幕坐标。
GLFWAPI void glfwSetCursorPos(GLFWwindow* window, double xpos, double ypos);
设置光标位置,窗口必须有输入焦点,如果没有,这个函数无作用。原点在左上角。
GLFWAPI GLFWcursor* glfwCreateCursor(const GLFWimage* image, int xhot, int yhot);
创建一个自定义的光标,结合set一起使用,光比较的照片存储和图标的方式一样,按照像素,从左上角开始。
GLFWAPI GLFWcursor* glfwCreateStandardCursor(int shape);
以标准形状创建一个光标
GLFWAPI void glfwDestroyCursor(GLFWcursor* cursor);
销毁一个光标
GLFWAPI void glfwSetCursor(GLFWwindow* window, GLFWcursor* cursor);
设置光标开始在窗口上进行使用,光标要显示害死哟啊设置光标的模式为GLFW_CURSOR_NORMAL。在一些平台上,如果已经有了输入焦点,这个光标就不会显示。
GLFWAPI int glfwJoystickPresent(int jid);
查询操纵杆是否存在
GLFWAPI const float* glfwGetJoystickAxes(int jid, int* count);
返回操纵杆所有轴的值,是一个数组,范围时-1到1
GLFWAPI const unsigned char* glfwGetJoystickButtons(int jid, int* count);
返回操纵杆的所有butto的状态,是一个数组,元素是按下或释放GLFW_PRESS or GLFW_RELEASE
GLFWAPI const unsigned char* glfwGetJoystickHats(int jid, int* count);
返回操纵杆所有hats的状态,返回的也是一个数组
GLFWAPI const char* glfwGetJoystickName(int jid);
返回操纵杆的名字,返回的是字符串
GLFWAPI const char* glfwGetJoystickGUID(int jid);
返回操纵杆的SDL兼容GUID,是一个UTF-8的16进制编码
GLFWAPI void glfwSetJoystickUserPointer(int jid, void* pointer);
操纵杆设置一个自动以指针值
GLFWAPI void* glfwGetJoystickUserPointer(int jid);
返回用户自定义的操纵杆指针值
GLFWAPI int glfwJoystickIsGamepad(int jid);
查询操纵杆是否在gamepad上进行过map
GLFWAPI int glfwUpdateGamepadMappings(const char* string);
添加SDL_GameControllerDB gamepad映射。
GLFWAPI const char* glfwGetGamepadName(int jid);
获取操纵杆游戏设备的名称
GLFWAPI int glfwGetGamepadState(int jid, GLFWgamepadstate* state);
获取游戏pad中操纵杆映射的状态,用在Xbox等游戏pad上。
GLFWAPI void glfwSetClipboardString(GLFWwindow* window, const char* string);
设置字符串的剪切板
GLFWAPI const char* glfwGetClipboardString(GLFWwindow* window);
获取剪切板的内容,返回的时字符串。
GLFWAPI GLFWwindow* glfwGetCurrentContext(void);
返回当前县城的GL/ES上下文
GLFWAPI int glfwExtensionSupported(const char* extension);
查询指定的GL/ES扩展是否支持
GLFWAPI GLFWglproc glfwGetProcAddress(const char* procname);
赶回当前上下文支持的GL/ES函数地址,包含核心库和扩展库。
callback函数:
GLFWAPI GLFWjoystickfun glfwSetJoystickCallback(GLFWjoystickfun callback);
设置操纵杆配置的回调函数,也可以覆盖原来的回调函数,当操纵杆连接或者断开连接的时候调用回调函数。回调函数的格式为:void function_name(int jid, int event)
GLFWAPI GLFWmonitorfun glfwSetMonitorCallback(GLFWmonitorfun callback);
设置monitor的毁掉函数,如果之前又设置,会替换之前的值。
GLFWAPI GLFWwindowposfun glfwSetWindowPosCallback(GLFWwindow* window, GLFWwindowposfun callback);
设置window的position callback,当窗口移动的时候会调用这个回调函数,返回的位置是屏幕坐标的,左上角是原点
GLFWAPI GLFWwindowsizefun glfwSetWindowSizeCallback(GLFWwindow* window, GLFWwindowsizefun callback);
设置窗口size的callback函数,当窗口resize的时候会调用,也是屏幕坐标。
GLFWAPI GLFWwindowclosefun glfwSetWindowCloseCallback(GLFWwindow* window, GLFWwindowclosefun callback);
设置窗口关闭的callback,窗口关闭阿的时候调用。
GLFWAPI GLFWwindowrefreshfun glfwSetWindowRefreshCallback(GLFWwindow* window, GLFWwindowrefreshfun callback);
设置窗口刷新的callback函数,刷新窗口的时候调用,if the window has been exposed after having been covered by another window.
GLFWAPI GLFWwindowfocusfun glfwSetWindowFocusCallback(GLFWwindow* window, GLFWwindowfocusfun callback);
设置窗口的focus回调函数,当窗口window gains or loses input focus时调用.
GLFWAPI GLFWwindowiconifyfun glfwSetWindowIconifyCallback(GLFWwindow* window, GLFWwindowiconifyfun callback);
设置iconification回调函数,窗口is iconified or restored时调用
GLFWAPI GLFWwindowmaximizefun glfwSetWindowMaximizeCallback(GLFWwindow* window, GLFWwindowmaximizefun callback);
设置窗口最大化的回调函数,窗口最大化或复位时调用
GLFWAPI GLFWframebuffersizefun glfwSetFramebufferSizeCallback(GLFWwindow* window, GLFWframebuffersizefun callback);
设置framebuffer resize的回调函数,当framebuffer resize时调用
GLFWAPI GLFWwindowcontentscalefun glfwSetWindowContentScaleCallback(GLFWwindow* window, GLFWwindowcontentscalefun callback);
设置窗口的content scale回调函数,当窗口的content scale变化时调用
GLFWAPI GLFWkeyfun glfwSetKeyCallback(GLFWwiunning a test, x11perf determinendow* window, GLFWkeyfun callback);
设置按键的回调函数,在按键按下、重复和释放时调用回调函数。
GLFWAPI GLFWcharfun glfwSetCharCallback(GLFWwindow* window, GLFWcharfun callback);
设置字符的回调函数,在输入指定字符编码时调用该回调函数。
GLFWAPI GLFWcharmodsfun glfwSetCharModsCallback(GLFWwindow* window, GLFWcharmodsfun callback);
设置字符串编码修改的回调函数。
GLFWAPI GLFWmousebuttonfun glfwSetMouseButtonCallback(GLFWwindow* window, GLFWmousebuttonfun callback);
设置鼠标按键的回调函数,鼠标按下或释放时触发,回调函数的格式:void function_name(GLFWwindow* window, int button, int action, int mods)
GLFWAPI GLFWcursorposfun glfwSetCursorPosCallback(GLFWwindow* window, GLFWcursorposfun callback);
设置光标位置回调函数,光标移动时触发。
GLFWAPI GLFWcursorenterfun glfwSetCursorEnterCallback(GLFWwindow* window, GLFWcursorenterfun callback);
设置光标进入离开的回调函数,当光标进入或离开屏幕指定区域时触发。回调函数的格式为:void function_name(GLFWwindow* window, int entered)
GLFWAPI GLFWscrollfun glfwSetScrollCallback(GLFWwindow* window, GLFWscrollfun callback);
设置滚轮回调函数
回调函数
void function_name(GLFWwindow* window, int path_count, const char* paths[])
GLFWAPI GLFWdropfun glfwSetDropCallback(GLFWwindow* window, GLFWdropfun callback);
设置path drop回调函数,当一个或多份额dragged paths are dropped时触发。
vulkan函数:
GLFWAPI int glfwVulkanSupported(void);
查询是否找到Vulkan loader and any minimally functional ICD
GLFWAPI const char** glfwGetRequiredInstanceExtensions(uint32_t* count);
返回GLFW需要的Vulkan instance extensions,返回的个数组,里面包含了,创建vulkan surface时GLFW要的Vulkan实例扩展。
GLFWAPI GLFWvkproc glfwGetInstanceProcAddress(VkInstance instance, const char* procname);
返回specified Vulkan instance函数地址
GLFWAPI int glfwGetPhysicalDevicePresentationSupport(VkInstance instance, VkPhysicalDevice device, uint32_t queuefamily);
查询当前GLFW编译的指定物理设备的queue family是否显示图像
GLFWAPI VkResult glfwCreateWindowSurface(VkInstance instance, GLFWwindow* window, const VkAllocationCallbacks* allocator, VkSurfaceKHR* surface);
创建一个surface。
最新版本:3.0
该版本移除了废弃的功能,同时增加了新的 API 用于支持多窗口和监视器,支持 sRGB、可靠性、OpenGL ES、high-DPI、gamma ramps、更多事件的回调、剪贴板文本 I/O,错误描述回调等等。
官方主页:http://www.glfw.org/
GLFW is an Open Source, multi-platform library for creating windows with OpenGL contexts and managing input and events. It is easy to integrate into existing applications and does not lay claim to the main loop.
在计算机图形学和游戏开发领域,GLFW(Graphics Library Framework)库是一个广受欢迎的开源库,它为开发者提供了创建和管理窗口、处理输入事件以及与 OpenGL、Vulkan 等图形 API 交互的便捷方式。其最初由 Marcus Geelnard 于 2002 年开发,旨在为 OpenGL 应用程序提供一个简单的跨平台窗口创建和事件处理框架。经过多年的发展,其已经成为一个功能强大、稳定且易于使用的库,支持 Windows、macOS、Linux 等多种主流操作系统。
主要特性
跨平台支持:GLFW 能够在不同的操作系统上运行,使得开发者可以编写一次代码,在多个平台上部署应用程序,大大提高了开发效率。
窗口管理:GLFW 提供了一系列函数来创建、销毁和管理窗口,包括设置窗口大小、位置、标题、图标等属性,还支持全屏模式和多显示器环境。
输入事件处理:GLFW 可以捕获键盘、鼠标、游戏手柄等输入设备的事件,如按键按下、释放、移动、滚轮滚动等,方便开发者实现交互功能。
OpenGL 和 Vulkan 集成:GLFW 与 OpenGL 和 Vulkan 图形 API 紧密集成,能够方便地创建上下文并进行图形渲染,无论是 2D 还是 3D 图形开发都能轻松应对。
高分辨率支持:支持高分辨率显示器,确保在 Retina 屏幕等设备上能够提供清晰、细腻的图像显示。
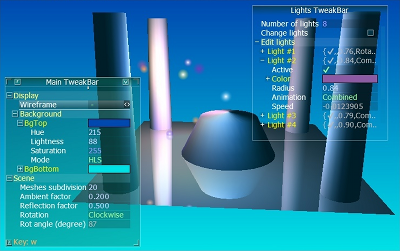
GLFW is written in C and has native support for Windows, Mac OS X and many Unix-like systems using the X Window System, such as Linux and FreeBSD. Licensed under the zlib/libpng license.
Features
Easy to use API in the style of the OpenGL APIs
Gives you a window and OpenGL context with two function calls
Explicit support for MSAA, sRGB, robustness, OpenGL 3.2+ and OpenGL ES
Support for multiple monitors, high-DPI, gamma ramps and clipboard
Input can be either polled or delivered via callbacks
UTF-8 for all strings and basic Unicode character input
Supports both static and dynamic linking
Open Source with a liberal OSI-certified license
Examples and comprehensive documentation
使用方法
在使用 GLFW 库之前,需要先将其安装到开发环境中。可以从 GLFW 官方网站下载源代码进行编译安装,也可以使用包管理器(如在 Linux 上使用 apt-get 或 yum,在 Windows 上使用 vcpkg)进行安装。基本用法如下:
使用 GLFW 库创建一个简单的窗口并显示,一般需要以下几个步骤:
1.初始化 GLFW 库:调用glfwInit()函数进行初始化。
2.创建窗口:使用glfwCreateWindow()函数创建一个窗口,并设置其大小、标题等属性。
3.创建 OpenGL 上下文:通过glfwMakeContextCurrent()函数将窗口的上下文设置为当前上下文。
4.事件循环:在一个循环中不断处理输入事件并进行渲染,直到用户关闭窗口。
5.清理资源:在程序结束时,调用glfwTerminate()函数释放 GLFW 库占用的资源。
应用场景
游戏开发:GLFW 库为游戏开发者提供了一个快速搭建游戏窗口和处理用户输入的基础框架,结合 OpenGL 或 Vulkan 等图形 API,可以创建出高性能的 2D 和 3D 游戏。
图形学研究:在计算机图形学的教学和研究中,GLFW 常用于创建图形演示程序,帮助学生和研究人员理解和实现各种图形算法。
虚拟现实(VR)和增强现实(AR)开发:GLFW 对多显示器和高分辨率的支持,使其成为 VR 和 AR 应用开发的理想选择,能够为用户提供沉浸式的体验。
GLFW 库以其简洁易用、功能强大和跨平台的特性,成为了图形开发领域不可或缺的工具。无论是初学者还是经验丰富的开发者,都可以通过 GLFW 库快速实现自己的图形应用程序,探索计算机图形学的无限可能。随着技术的不断发展,相信 GLFW 库会在更多的领域发挥重要作用。
GLAD
因为OpenGL只是一个标准/规范,具体的实现是由驱动开发商针对特定显卡实现的。由于OpenGL驱动版本众多,它大多数函数的位置都无法在编译时确定下来,需要在运行时查询。所以任务就落在了开发者身上,开发者需要在运行时获取函数地址并将其保存在一个函数指针中供以后使用,取得地址的方法因平台而异。
Vulkan/GL/GLES/EGL/GLX/WGL Loader-Generator based on the official specifications for multiple languages.
GLAD是一个开源的库,它能解决我们上面提到的那个平台问题。其配置与大多数的开源库有些许的不同,GLAD使用了一个在线服务。在这里能够告诉GLAD需要定义的OpenGL版本,并且根据这个版本加载所有相关的OpenGL函数。在其在线服务页面中将语言(Language)设置为C/C++,在API选项中,选择3.3以上的OpenGL(gl)版本(不少教程中将使用3.3版本,但更新的版本也能用)。之后将模式(Profile)设置为Core,并且保证选中了生成加载器(Generate a loader)选项。现在可以先(暂时)忽略扩展(Extensions)中的内容。都选择完之后,点击生成(Generate)按钮来生成库文件。稍后会应该提供给了一个zip压缩文件,包含两个头文件目录,和一个glad.c文件。将两个头文件目录(glad和KHR)复制到你的Include文件夹中(或者增加一个额外的项目指向这些目录),并添加glad.c文件到你的工程中。
经过前面的这些步骤之后,你就应该可以将以下的指令加到你的文件顶部了:
#include <glad/glad.h>
可以阅读Getting started来理解GLFW3的使用,glfw/examples目录下有数十个例子可以参考。
使用步骤:
首先包含头头文件
#include <glad/glad.h>
// GLFW (include after glad)
#include <GLFW/glfw3.h>
初始化glfw库:
在glfw使用之前先要出世,初始化只要是检查环境是不是支持。
GLFWAPI int glfwInit(void);
初始化GLFW库,在应用称许执行之前要先调用这个函数,对应的终止函数是glfwTerminate,在应用程序结束之前要调用glfwTerminate,成功返回GLFW_TRUE
设置错误回调:
GLFWAPI GLFWerrorfun glfwSetErrorCallback(GLFWerrorfun callback);
设置错误回调函数,当一个错误发生时回调用错误码和一段描述信息。回调函数的个是如下:
void error_callback(int error, const char* description){
fprintf(stderr, "Error: %s\n", description);
}
创建窗口和上下文:
GLFWAPI void glfwWindowHint(int hint, int value);
GLFWAPI void glfwWindowHintString(int hint, const char* value);
设置window的hints值,在glfwCreatewindow时生效,设置之后不会改变,知道遇到函数glfwDefaultWindowHints或GLFW终止。这个函数只能设置整形值,字符串的值通过glfwWindowHintString来设置。另外它不检查设置的值是否有效,在glfwCreateWindow的时候才会报。
GLFWAPI GLFWwindow* glfwCreateWindow(int width, int height, const char* title, GLFWmonitor* monitor, GLFWwindow* share);
创建GL/ES的上下文,参数指定了上下文如何创建,成功创建不影响当前上下文。
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 2);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 0);
GLFWwindow* window = glfwCreateWindow(640, 480, "My Title", NULL, NULL);
if (!window){
// Window or context creation failed
}
设置上下文:
GLFWAPI void glfwMakeContextCurrent(GLFWwindow* window);
指定当前GL/ES上下文
检查窗口的关闭标识:
GLFWAPI int glfwWindowShouldClose(GLFWwindow* window);
检查指定window的关闭状态.
while ( glfwWindowShouldClose(window) ){
//keep running
}
接收输入事件:
先设置事件回调函数,输入事件比较多。
static void key_callback(GLFWwindow* window, int key, int scancode, int action, int mods){
if (key == GLFW_KEY_ESCAPE && action == GLFW_PRESS)
glfwSetWindowShouldClose(window, GLFW_TRUE);
}
glfwSetKeyCallback(window, key_callback);
进行渲染:
渲染的时候framebuffer的size可以设置和viewport一样大,也可以设置framebuffer size的回调函数。
int width, height;
glfwGetFramebufferSize(window, &width, &height);
glViewport(0, 0, width, height);
时间读取:
GLFWAPI double glfwGetTime(void);
获取GLFW的时间,以秒为单位
GLFWAPI void glfwSetTime(double time);
设置GLFW的时间,以秒为单位,小于等于18446744073.0
GLFWAPI uint64_t glfwGetTimerValue(void);
获取raw timer的值,单位1 / frequency seconds
GLFWAPI uint64_t glfwGetTimerFrequency(void);
获取帧率单位是Hz
缓冲区交换:
GLFWAPI void glfwSwapBuffers(GLFWwindow* window);
交换窗口的前后缓冲区,如果交换间隔大于0,交换缓冲区之前GPU驱动会等待specified number of screen updates
GLFWAPI void glfwSwapInterval(int interval);
设置当前上下文的交换间隔
事件处理:
有两种处理未决事件的方法; 轮询和等待。
GLFWAPI void glfwPollEvents(void);
处理所有事件队列中的事件,事件处理会调用一些回调函数。一些平台下,窗口移动、resize和菜单操作都会触发事件处理,block当前的任务。
GLFWAPI void glfwWaitEvents(void);
等待所有处理中和队列中的事件完成,当前线程会进入sleep状态,如果有事件可用,就相当与glfwPollEvents.
销毁和注销:
GLFWAPI void glfwTerminate(void);
这个函数终止GLFW,这个函数会注销调剩余的windows and cursors,复位所有修改过的gamma ramps,释放申请的资源。对应glfwInit
其他API
GLFWAPI void glfwInitHint(int hint, int value);
设置制定的初始化hint值,这个设置之后中间不能进行修改直至结束。有些hints是平台相关,这些只有在对应的平台上才会生效。
GLFWAPI void glfwGetVersion(int* major, int* minor, int* rev);
查询GLFW库的版本,major, minor and revision号
GLFWAPI const char* glfwGetVersionString(void);
查询编译阶段的配置,返回的是个字符串,包含版本、平台、编译器和平台相关的编译阶段的选项
GLFWAPI int glfwGetError(const char** description);
查询错误码并清除上一次的错误信息
GLFWAPI GLFWmonitor** glfwGetMonitors(int* count);
查询当前的连接监视器connected monitors,返回的是当前所有连接的monitor,primary monitor永远是数组的第一个。
GLFWAPI GLFWmonitor* glfwGetPrimaryMonitor(void);
查询primary monitor,task bar和global menu bar等基本信息都是显示在primary monitor中的。
GLFWAPI void glfwGetMonitorPos(GLFWmonitor* monitor, int* xpos, int* ypos);
获取虚拟屏幕中monitor的视口坐标
GLFWAPI void glfwGetMonitorWorkarea(GLFWmonitor* monitor, int* xpos, int* ypos, int* width, int* height);
获取monitor的工作区,返回的是屏幕坐标的位置,左上角和size的值。工作区是屏幕中没有系统任务条的区域,如果系统没有任务条,就是全屏幕。
GLFWAPI void glfwGetMonitorPhysicalSize(GLFWmonitor* monitor, int* widthMM, int* heightMM);
获取monitor的物理尺寸,单位是millimetres
GLFWAPI void glfwGetMonitorContentScale(GLFWmonitor* monitor, float* xscale, float* yscale);
获取monitor的content scale,这个值的计算:当前DPI与平台默认DPI之间的比。依赖于monitor的分辨率和设置的像素密度。
GLFWAPI const char* glfwGetMonitorName(GLFWmonitor* monitor);
获取monitor的名称
GLFWAPI void glfwSetMonitorUserPointer(GLFWmonitor* monitor, void* pointer);
设置monitor中用户自定义的pointer
GLFWAPI void* glfwGetMonitorUserPointer(GLFWmonitor* monitor);
获取monitor的用户自定义pointer
GLFWAPI const GLFWvidmode* glfwGetVideoModes(GLFWmonitor* monitor, int* count);
获取monitor的可用视频模式,返回的是一个数组,按照升序排列。
GLFWAPI const GLFWvidmode* glfwGetVideoMode(GLFWmonitor* monitor);
获取monitor的当前的视频模式,如果是全屏模式,返回值以来窗口是否iconified
GLFWAPI void glfwSetGamma(GLFWmonitor* monitor, float gamma);
根据指定的exponent生成一个合适尺寸的gamma ramp(gamma斜度)并设置,参数必须是一个大于0的finite number,用于色彩矫正,理想值是1.
GLFWAPI const GLFWgammaramp* glfwGetGammaRamp(GLFWmonitor* monitor);
获取当前的gamma ramp值
GLFWAPI void glfwSetGammaRamp(GLFWmonitor* monitor, const GLFWgammaramp* ramp);
设置monitor的gamma ramp,第一次调用这个函数并存储gamma的值是在glfwTerminate函数中
GLFWAPI void glfwDefaultWindowHints(void);
reset窗口的所有hints值,全设置为默认的。
GLFWAPI void glfwDestroyWindow(GLFWwindow* window);
destory指定的window和context
GLFWAPI void glfwSetWindowShouldClose(GLFWwindow* window, int value);
设置window的close flag。
GLFWAPI void glfwSetWindowTitle(GLFWwindow* window, const char* title);
设置窗口的title
GLFWAPI void glfwSetWindowIcon(GLFWwindow* window, int count, const GLFWimage* images);
为窗口设置Icon,传入一组备选图像,会选择尺寸接近的作为图标,如果没有传图像,用默认的图标。图像是32bit小端non-premultiplied RGBA8,按行排列从左上角开始。图像的尺寸会根据平台和系统设置进行resize,一般包括16x16,32x32和48x48.
GLFWAPI void glfwGetWindowPos(GLFWwindow* window, int* xpos, int* ypos);
获取指定区域的位置,返回的是屏幕坐标,原点在左上角
GLFWAPI void glfwSetWindowPos(GLFWwindow* window, int xpos, int ypos);
设置窗口坐标,如果是全屏没有效果
GLFWAPI void glfwGetWindowSize(GLFWwindow* window, int* width, int* height);
获取窗口的size,以屏幕坐标为单位,不是framebuffer的尺寸。
GLFWAPI void glfwSetWindowSizeLimits(GLFWwindow* window, int minwidth, int minheight, int maxwidth, int maxheight);
设置窗口的尺寸限制,如果是全屏模式,这个参数只有在创建窗口的时候其作用。其他情况下窗口要是可缩放的这个函数才有作用。
GLFWAPI void glfwSetWindowAspectRatio(GLFWwindow* window, int numer, int denom);
设置窗口的aspect ratio,如果是全屏模式,这个参数只有在创建窗口的时候其作用。其他情况下窗口要是可缩放的这个函数才有作用。aspect ratios是长宽比要大于0。
GLFWAPI void glfwSetWindowSize(GLFWwindow* window, int width, int height);
设置窗口的size.对于全屏窗口,此功能可更新其所需视频模式的分辨率并切换到最接近其的视频模式,而不会影响窗口的上下文。 由于上下文不受影响,因此帧缓冲区的位深度保持不变.
GLFWAPI void glfwGetFramebufferSize(GLFWwindow* window, int* width, int* height);
获取窗口的framebuffer的zise,以pixel为单位
GLFWAPI void glfwGetWindowFrameSize(GLFWwindow* window, int* left, int* top, int* right, int* bottom);
以屏幕坐标为单位检索指定窗口框架的每个边缘的大小。包括标题栏。函数检索每个窗框边缘的大小,而不是沿特定坐标轴的偏移量,所以检索到的值将始终为零或正数。
GLFWAPI void glfwGetWindowContentScale(GLFWwindow* window, float* xscale, float* yscale);
此函数检索指定窗口的内容比例。 内容比例是当前DPI与平台默认DPI之比。 这对于文本和任何UI元素尤其重要。
GLFWAPI float glfwGetWindowOpacity(GLFWwindow* window);
获取窗苦的不透明度(alpha值),包括所有装饰物。该值时0到1之间的值,初始值为1
GLFWAPI void glfwSetWindowOpacity(GLFWwindow* window, float opacity);
设置窗口的透明度
GLFWAPI void glfwIconifyWindow(GLFWwindow* window);
如果指定的窗口先前已还原,则此功能可图标化(最小化)指定的窗口。如果窗口已被图标化,则此功能不执行任何操作。如果指定的窗口是全屏窗口,则将还原原始监视器分辨率,直到还原该窗口为止。
GLFWAPI void glfwRestoreWindow(GLFWwindow* window);
重置窗口,用在窗口进行了最大化或最小化的情况。如果指定的窗口是全屏窗口,则为该窗口选择的分辨率将在所选监视器上恢复。
GLFWAPI void glfwMaximizeWindow(GLFWwindow* window);
最大化窗口,如果窗口已经最大化,或是全屏模式不起作用
GLFWAPI void glfwShowWindow(GLFWwindow* window);
显示窗口,全屏不起作用,默认情况所有创建的窗口都是显示的,也可以通过glfwSetWindowAttrib设置GLFW_FOCUS_ON_SHOW_hint来设置
GLFWAPI void glfwHideWindow(GLFWwindow* window);
隐藏窗口,全屏不起作用
GLFWAPI void glfwFocusWindow(GLFWwindow* window);
此功能将指定的窗口置于最前面并设置输入焦点。 该窗口应该已经可见并且没有图标。默认情况下,窗口和全屏模式窗口在最初创建时都会被聚焦。GLFWAPI void glfwRequestWindowAttention(GLFWwindow* window);
该功能要求用户注意指定的窗口。 作用不详
GLFWAPI GLFWmonitor* glfwGetWindowMonitor(GLFWwindow* window);
返回指定窗口处于全屏状态的监视器的句柄
GLFWAPI void glfwSetWindowMonitor(GLFWwindow* window, GLFWmonitor* monitor, int xpos, int ypos, int width, int height, int refreshRate);
设置指定窗口处于全屏状态的监视器的句柄,这个函数会更新monitor的宽、高、视频模式的刷新率。
GLFWAPI int glfwGetWindowAttrib(GLFWwindow* window, int attrib);
获取窗口或GL/ES上下文相关的属性值,framebuffer相关的hints不是属性。
GLFWAPI void glfwSetWindowAttrib(GLFWwindow* window, int attrib, int value);
设置窗口的属性值,支持的属性包含:GLFW_DECORATED、GLFW_RESIZABLE、GLFW_FLOATING、GLFW_AUTO_ICONIFY、GLFW_FOCUS_ON_SHOW。
GLFWAPI void glfwSetWindowUserPointer(GLFWwindow* window, void* pointer);
设置window的user pointer
GLFWAPI void* glfwGetWindowUserPointer(GLFWwindow* window);
返回window的user pointer
GLFWAPI void glfwWaitEventsTimeout(double timeout);
等待超时。
GLFWAPI void glfwPostEmptyEvent(void);
向事件队列中插入一个空事件
GLFWAPI int glfwGetInputMode(GLFWwindow* window, int mode);
获取窗口输入选项的值,mode取值为:GLFW_CURSOR, GLFW_STICKY_KEYS, GLFW_STICKY_MOUSE_BUTTONS, GLFW_LOCK_KEY_MODS, GLFW_RAW_MOUSE_MOTION。
GLFWAPI void glfwSetInputMode(GLFWwindow* window, int mode, int value);
设置窗口的输入模式,mode取值和get函数一样。
GLFWAPI int glfwRawMouseMotionSupported(void);
查询当前系统是否支持raw mouse motion,是否支持在初始化GLFW的时候一次确定,raw motion接近actual motion,它不受应用于桌面光标运动的缩放和加速度的影响。 该处理适合于光标,而原始运动更适合于控制3D相机。因此,仅在禁用光标时才提供原始鼠标移动。
GLFWAPI const char* glfwGetKeyName(int key, int scancode);
获取按键名。
GLFWAPI int glfwGetKeyScancode(int key);
获取按键码。
GLFWAPI int glfwGetKey(GLFWwindow* window, int key);
获取按键的最后一个状态。
GLFWAPI int glfwGetMouseButton(GLFWwindow* window, int button);
获取鼠标的最后状态值。
GLFWAPI void glfwGetCursorPos(GLFWwindow* window, double* xpos, double* ypos);
获取光标位置,屏幕坐标。
GLFWAPI void glfwSetCursorPos(GLFWwindow* window, double xpos, double ypos);
设置光标位置,窗口必须有输入焦点,如果没有,这个函数无作用。原点在左上角。
GLFWAPI GLFWcursor* glfwCreateCursor(const GLFWimage* image, int xhot, int yhot);
创建一个自定义的光标,结合set一起使用,光比较的照片存储和图标的方式一样,按照像素,从左上角开始。
GLFWAPI GLFWcursor* glfwCreateStandardCursor(int shape);
以标准形状创建一个光标
GLFWAPI void glfwDestroyCursor(GLFWcursor* cursor);
销毁一个光标
GLFWAPI void glfwSetCursor(GLFWwindow* window, GLFWcursor* cursor);
设置光标开始在窗口上进行使用,光标要显示害死哟啊设置光标的模式为GLFW_CURSOR_NORMAL。在一些平台上,如果已经有了输入焦点,这个光标就不会显示。
GLFWAPI int glfwJoystickPresent(int jid);
查询操纵杆是否存在
GLFWAPI const float* glfwGetJoystickAxes(int jid, int* count);
返回操纵杆所有轴的值,是一个数组,范围时-1到1
GLFWAPI const unsigned char* glfwGetJoystickButtons(int jid, int* count);
返回操纵杆的所有butto的状态,是一个数组,元素是按下或释放GLFW_PRESS or GLFW_RELEASE
GLFWAPI const unsigned char* glfwGetJoystickHats(int jid, int* count);
返回操纵杆所有hats的状态,返回的也是一个数组
GLFWAPI const char* glfwGetJoystickName(int jid);
返回操纵杆的名字,返回的是字符串
GLFWAPI const char* glfwGetJoystickGUID(int jid);
返回操纵杆的SDL兼容GUID,是一个UTF-8的16进制编码
GLFWAPI void glfwSetJoystickUserPointer(int jid, void* pointer);
操纵杆设置一个自动以指针值
GLFWAPI void* glfwGetJoystickUserPointer(int jid);
返回用户自定义的操纵杆指针值
GLFWAPI int glfwJoystickIsGamepad(int jid);
查询操纵杆是否在gamepad上进行过map
GLFWAPI int glfwUpdateGamepadMappings(const char* string);
添加SDL_GameControllerDB gamepad映射。
GLFWAPI const char* glfwGetGamepadName(int jid);
获取操纵杆游戏设备的名称
GLFWAPI int glfwGetGamepadState(int jid, GLFWgamepadstate* state);
获取游戏pad中操纵杆映射的状态,用在Xbox等游戏pad上。
GLFWAPI void glfwSetClipboardString(GLFWwindow* window, const char* string);
设置字符串的剪切板
GLFWAPI const char* glfwGetClipboardString(GLFWwindow* window);
获取剪切板的内容,返回的时字符串。
GLFWAPI GLFWwindow* glfwGetCurrentContext(void);
返回当前县城的GL/ES上下文
GLFWAPI int glfwExtensionSupported(const char* extension);
查询指定的GL/ES扩展是否支持
GLFWAPI GLFWglproc glfwGetProcAddress(const char* procname);
赶回当前上下文支持的GL/ES函数地址,包含核心库和扩展库。
callback函数:
GLFWAPI GLFWjoystickfun glfwSetJoystickCallback(GLFWjoystickfun callback);
设置操纵杆配置的回调函数,也可以覆盖原来的回调函数,当操纵杆连接或者断开连接的时候调用回调函数。回调函数的格式为:void function_name(int jid, int event)
GLFWAPI GLFWmonitorfun glfwSetMonitorCallback(GLFWmonitorfun callback);
设置monitor的毁掉函数,如果之前又设置,会替换之前的值。
GLFWAPI GLFWwindowposfun glfwSetWindowPosCallback(GLFWwindow* window, GLFWwindowposfun callback);
设置window的position callback,当窗口移动的时候会调用这个回调函数,返回的位置是屏幕坐标的,左上角是原点
GLFWAPI GLFWwindowsizefun glfwSetWindowSizeCallback(GLFWwindow* window, GLFWwindowsizefun callback);
设置窗口size的callback函数,当窗口resize的时候会调用,也是屏幕坐标。
GLFWAPI GLFWwindowclosefun glfwSetWindowCloseCallback(GLFWwindow* window, GLFWwindowclosefun callback);
设置窗口关闭的callback,窗口关闭阿的时候调用。
GLFWAPI GLFWwindowrefreshfun glfwSetWindowRefreshCallback(GLFWwindow* window, GLFWwindowrefreshfun callback);
设置窗口刷新的callback函数,刷新窗口的时候调用,if the window has been exposed after having been covered by another window.
GLFWAPI GLFWwindowfocusfun glfwSetWindowFocusCallback(GLFWwindow* window, GLFWwindowfocusfun callback);
设置窗口的focus回调函数,当窗口window gains or loses input focus时调用.
GLFWAPI GLFWwindowiconifyfun glfwSetWindowIconifyCallback(GLFWwindow* window, GLFWwindowiconifyfun callback);
设置iconification回调函数,窗口is iconified or restored时调用
GLFWAPI GLFWwindowmaximizefun glfwSetWindowMaximizeCallback(GLFWwindow* window, GLFWwindowmaximizefun callback);
设置窗口最大化的回调函数,窗口最大化或复位时调用
GLFWAPI GLFWframebuffersizefun glfwSetFramebufferSizeCallback(GLFWwindow* window, GLFWframebuffersizefun callback);
设置framebuffer resize的回调函数,当framebuffer resize时调用
GLFWAPI GLFWwindowcontentscalefun glfwSetWindowContentScaleCallback(GLFWwindow* window, GLFWwindowcontentscalefun callback);
设置窗口的content scale回调函数,当窗口的content scale变化时调用
GLFWAPI GLFWkeyfun glfwSetKeyCallback(GLFWwiunning a test, x11perf determinendow* window, GLFWkeyfun callback);
设置按键的回调函数,在按键按下、重复和释放时调用回调函数。
GLFWAPI GLFWcharfun glfwSetCharCallback(GLFWwindow* window, GLFWcharfun callback);
设置字符的回调函数,在输入指定字符编码时调用该回调函数。
GLFWAPI GLFWcharmodsfun glfwSetCharModsCallback(GLFWwindow* window, GLFWcharmodsfun callback);
设置字符串编码修改的回调函数。
GLFWAPI GLFWmousebuttonfun glfwSetMouseButtonCallback(GLFWwindow* window, GLFWmousebuttonfun callback);
设置鼠标按键的回调函数,鼠标按下或释放时触发,回调函数的格式:void function_name(GLFWwindow* window, int button, int action, int mods)
GLFWAPI GLFWcursorposfun glfwSetCursorPosCallback(GLFWwindow* window, GLFWcursorposfun callback);
设置光标位置回调函数,光标移动时触发。
GLFWAPI GLFWcursorenterfun glfwSetCursorEnterCallback(GLFWwindow* window, GLFWcursorenterfun callback);
设置光标进入离开的回调函数,当光标进入或离开屏幕指定区域时触发。回调函数的格式为:void function_name(GLFWwindow* window, int entered)
GLFWAPI GLFWscrollfun glfwSetScrollCallback(GLFWwindow* window, GLFWscrollfun callback);
设置滚轮回调函数
回调函数
void function_name(GLFWwindow* window, int path_count, const char* paths[])
GLFWAPI GLFWdropfun glfwSetDropCallback(GLFWwindow* window, GLFWdropfun callback);
设置path drop回调函数,当一个或多份额dragged paths are dropped时触发。
vulkan函数:
GLFWAPI int glfwVulkanSupported(void);
查询是否找到Vulkan loader and any minimally functional ICD
GLFWAPI const char** glfwGetRequiredInstanceExtensions(uint32_t* count);
返回GLFW需要的Vulkan instance extensions,返回的个数组,里面包含了,创建vulkan surface时GLFW要的Vulkan实例扩展。
GLFWAPI GLFWvkproc glfwGetInstanceProcAddress(VkInstance instance, const char* procname);
返回specified Vulkan instance函数地址
GLFWAPI int glfwGetPhysicalDevicePresentationSupport(VkInstance instance, VkPhysicalDevice device, uint32_t queuefamily);
查询当前GLFW编译的指定物理设备的queue family是否显示图像
GLFWAPI VkResult glfwCreateWindowSurface(VkInstance instance, GLFWwindow* window, const VkAllocationCallbacks* allocator, VkSurfaceKHR* surface);
创建一个surface。
最新版本:3.0
该版本移除了废弃的功能,同时增加了新的 API 用于支持多窗口和监视器,支持 sRGB、可靠性、OpenGL ES、high-DPI、gamma ramps、更多事件的回调、剪贴板文本 I/O,错误描述回调等等。
官方主页:http://www.glfw.org/